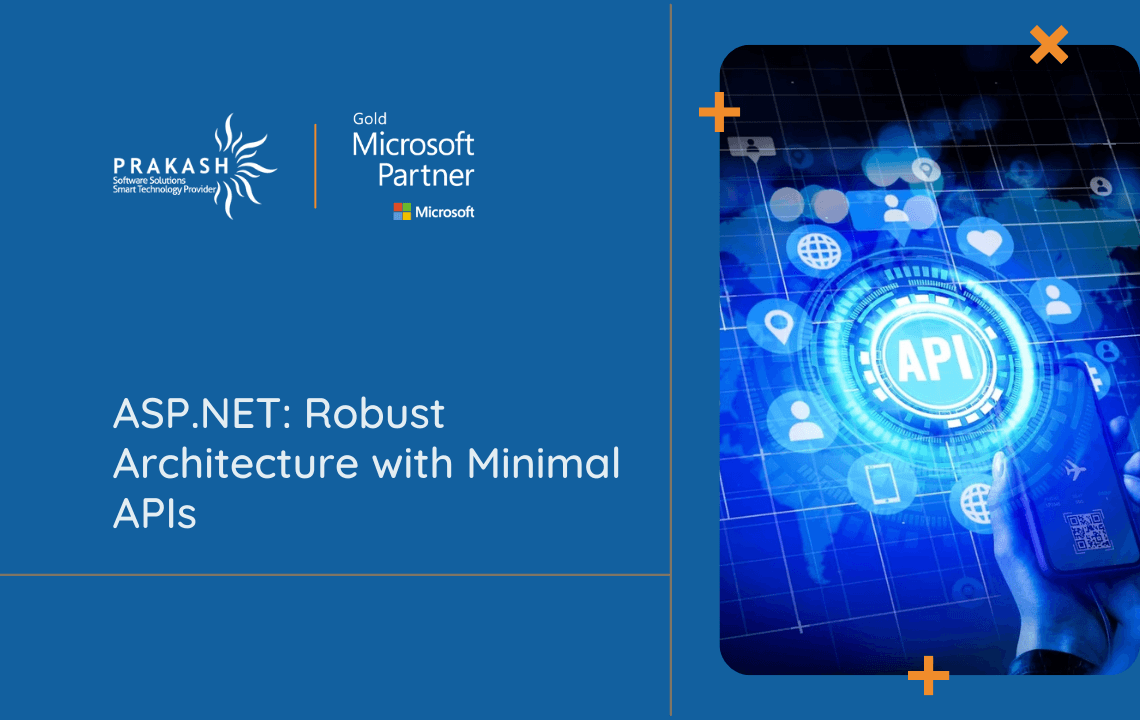
ASP.NET: Robust Architecture with Minimal APIs
In the realm of ASP.NET development, adhering to sound architectural practices is paramount for ensuring the maintainability, flexibility, and separation of concerns within software projects. With the emergence of DOTNET 7 and its Minimal APIs, developers have a powerful tool at their disposal to streamline the construction of Web API projects while maintaining architectural integrity.
One key aspect of a robust architecture involves the implementation of service-controller separation, were distinct layers handle business logic and HTTP request processing independently.
By adhering to this principle, developers can enhance the project’s structure and scalability, facilitating easier maintenance and future expansions.
Additionally, it is essential to differentiate between entity models, representing data structures within the application, and view models, which cater to the specific needs of client interactions. This clear distinction ensures that the API’s data handling remains organized and adaptable to changing requirements.
In this blog post, we delve into the process of implementing such an architecture within a DOTNET 7 Web API project. We’ll explore how Minimal APIs, with their streamlined approach to defining endpoints, complement the service-controller separation paradigm.
Furthermore, we’ll discuss the benefits of utilizing minimal API mapgroup to efficiently map between entity models and view models, promoting code reusability and reducing development overhead.
Leveraging the capabilities of minimal API mapgroup, developers can construct APIs that are adaptable to evolving business needs.
Let’s get started.
What are Minimal APIs?
In previous iterations of .NET, creating an API to facilitate communication between different components of your application typically involved the use of “controllers.”
These controllers acted as intermediaries, akin to traffic cops, handling incoming requests, processing them, and generating appropriate responses.
With the introduction of .NET 7, a new concept called “Minimal APIs” has been introduced. Think of Minimal APIs as a simplified alternative to traditional controllers for your APIs. Instead of grappling with complex code to configure controllers and actions, Minimal APIs offer a more straightforward approach.
Simplified API Creation with Minimal APIs
Consider the scenario where you need to create an API to retrieve information about books. With Minimal APIs, you can accomplish this task with just a few lines of code, bypassing the need to set up an entire controller with methods and routes.
This approach is more direct and minimalist, speeding up the development process. If you’re accustomed to working with controllers in MVC, think of Minimal APIs as a faster and simpler way to construct APIs without the overhead of extensive setup.
This streamlined approach accelerates the creation of communication points within your application, expediting the development cycle.
One notable advantage of Minimal APIs over controllers is their conciseness. Controllers often entail additional tasks beyond data transmission, such as user authentication, input validation, and error handling, leading to verbose code.
In contrast, Minimal APIs typically consist of just a few lines of code, reducing complexity and enhancing readability. In summary, Minimal APIs represent a quicker and more efficient means of building APIs compared to traditional controllers.
By embracing Minimal APIs, developers can streamline the development process and expedite the deployment of communication endpoints within their applications.
As an illustration, consider the subsequent code snippet, which demonstrates the creation of a controller action responsible for returning a list of employees. While this code is relatively straightforward, it necessitates several lines of code to authenticate the user, validate input, and handle potential errors.
[HttpGet] public IActionResult GetEmployees()
{
return Ok(db.Employees.ToList()); }
In contrast, observe the following code snippet, showcasing the creation of a minimal API designed to retrieve a list of employees.
app.MapGet("/employees", () => db.Employees.ToList());
The code provided offers a concise and simplified alternative to traditional controller-based approaches. Unlike controller actions, which require authentication, validation, and error handling, the presented minimal API requires none of these additional complexities, making it ideal for straightforward and lightweight API implementations.
Some Important considerations and common misconceptions regarding minimal APIs:
- Less Flexibility: While controllers are often tailored to specific data or operations, minimal APIs offer greater flexibility, allowing them to handle a wider range of tasks.
- Difficulty in Testing: Controllers can be challenging to test in isolation due to their dependencies on other parts of the application, such as databases or user interfaces. In contrast, minimal APIs are more easily testable as they can function independently of other components.
- Maintenance Challenges: Controllers can become intricate and difficult to maintain over time, especially when responsible for multiple operations. In contrast, minimal APIs, focusing on single operations, are typically easier to manage.
Misconceptions
- Not a Full-Featured Web Framework: Minimal APIs provide the essentials for building RESTful APIs and can be augmented with additional features as needed.
- Suitability for Large Projects: While minimal APIs excel in simplicity, they can also be employed in large and complex projects when designed and implemented thoughtfully.
- Ease of Learning: Despite some misconceptions, minimal APIs are relatively easy to learn and use, with concise and readable code.
- Security: Minimal APIs are inherently secure due to default security features like HTTPS, but developers should still follow best practices for secure development.
Regarding file organization, while some may mistakenly believe that all code must reside in a single file like Program.cs, utilizing extension methods allows for a more organized structure. By employing extension methods, developers can modularize their codebase, enhancing readability and maintainability.
Moving forward, the architecture of minimal APIs for large-scale projects can be organized using extension methods to split endpoints across multiple files. This approach diverges from the traditional controller-based structure, offering flexibility and scalability.
Steps for implementation
- Setting up the application,
- Extending the Web Application,
- Defining endpoints,
- Simplifying endpoint registration,
- Defining model classes, and
- Understanding key concepts like ResultsWrapper and RouteGroupBuilderExtensions.
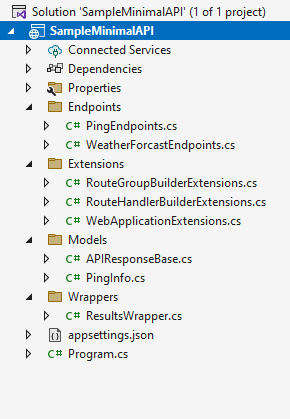
Step 1: Setting up the application
To initiate the application, the process begins with the SampleMinimalAPI/Program.cs file, serving as the entry point. Below is an excerpt showcasing the structure of the code:
using SampleMinimalAPI.Extensions;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.RegisterEndpoints();
app.UseHttpsRedirection();
app.Run();
Explanation: The builder is utilized for constructing the application. In case the application operates within the development environment, Swagger and SwaggerUI functionalities are enabled. Swagger facilitates the documentation of API endpoints.
The RegisterEndpoints() method is invoked to enlist the API endpoints. Further elaboration on this method will be provided in Step 2 of this blog.
Step 2: Extending the WebApplication
Contained within the SampleMinimalAPI/Extensions/WebApplicationExtensions.cs file is an extension method designated for endpoint registration:
using SampleMinimalAPI.Endpoints;
namespace SampleMinimalAPI.Extensions;
public static class WebApplicationExtensions
{
public static void RegisterEndpoints(this WebApplication application)
{
var globalGroupV1 = application.MapGroup("api");
PingEndpoints.Endpoints(globalGroupV1);
WeatherForcastEndpoints.Endpoints(globalGroupV1);
}
}
Explanation: The RegisterEndpoints extension method is introduced for the WebApplication class to enhance the organization of endpoint registration.
Utilizing MapGroup, a global group labeled “api” is established.
Both PingEndpoints and WeatherForecastEndpoints are enlisted within the “api” group.
Step 3: Creating Ping Endpoints
Within the SampleMinimalAPI/Endpoints/PingEndpoints.cs file, the ping endpoint is defined:
using SampleMinimalAPI.Extensions;
using SampleMinimalAPI.Models;
using SampleMinimalAPI.Wrappers;
namespace SampleMinimalAPI.Endpoints;
public class PingEndpoints
{
public static void Endpoints(RouteGroupBuilder builder)
{
var group = builder.MapGroup("ping");
group.GetAnonymous("ping", PingV1)
.AddMetaDataAnonymous<PingInfo>();
}
internal static IResult PingV1()
{
// ... code to handle ping request and log the response
return ResultsWrapper.Ok(pingInfo);
}
}
Explanation: Within the PingEndpoints class, a static method named Endpoints is defined to facilitate the registration of ping endpoints.
Utilizing MapGroup, a group titled “ping” is established. An anonymous GET endpoint labeled “ping” is appended to the “ping” group.
The PingV1 method is responsible for managing the logic associated with the ping request. It logs a response and returns an “OK” result containing ping information.
Step 4: Creating Weather Forecast Endpoints
Contained within the SampleMinimalAPI/Endpoints/WeatherForcastEndpoints.cs file, weather forecast endpoints are defined:
using SampleMinimalAPI.Extensions;
using SampleMinimalAPI.Models;
using SampleMinimalAPI.Wrappers;
namespace SampleMinimalAPI.Endpoints;
public static class WeatherForcastEndpoints
{
public static void Endpoints(RouteGroupBuilder builder)
{
var group = builder.MapGroup("weather");
group.GetAnonymous("weatherforecast", GetWeatherForecast)
.AddMetaDataAnonymous<PingInfo>();
}
internal static IResult GetWeatherForecast()
{
// ... code to generate weather forecast data ...
return ResultsWrapper.Ok(forecast);
}
}
Explanation: Within the WeatherForcastEndpoints class, a static method named Endpoints is defined to facilitate the registration of weather forecast endpoints.
Utilizing MapGroup, a group labeled “weather” is established.
An anonymous GET endpoint titled “weatherforecast” is appended to the “weather” group.
The GetWeatherForecast method is responsible for producing weather forecast data and returning an “Ok” result containing the forecast.
Step 5: Simplifying Endpoint Registration
Contained within the SampleMinimalAPI/Extensions/RouteGroupBuilderExtensions.cs file are extension methods devised to streamline endpoint registration:
namespace SampleMinimalAPI.Extensions;
public static class RouteGroupBuilderExtensions
{
public static RouteHandlerBuilder Get(this RouteGroupBuilder webApplication, string route, Delegate action, string routeName = null)
{
// ... logic to register a GET route ...
}
// ... similar extension methods for POST, PUT, DELETE, and anonymous routes ...
}
Explanation: Extension methods are defined to incorporate common HTTP routes using MapGet, MapPost, MapPut, and MapDelete.
Additionally, there are extension methods designated for anonymous routes.
These methods contribute to the succinctness and clarity of endpoint registration.
Step 6: Defining Model Classes
Model classes are established to structure the responses of the API:
- APIResponseBase serves as a generic class for API responses, encompassing elements such as status code, success status, message, and result.
- PingInfo embodies the response data for the ping endpoint.
- WeatherForecast represents weather forecast data as a record.
Step 7: Understanding ResultsWrapper and RouteGroupBuilderExtensions
- ResultsWrapper serves as a utility tool simplifying the creation of API responses by encapsulating data in a standardized format, inclusive of status codes and success indicators.
- RouteGroupBuilderExtensions furnishes convenient extension methods for registering various types of routes (GET, POST, PUT, DELETE) and anonymous routes. These methods enhance code readability and diminish redundancy during endpoint registration.
Note: A functional example is available on GitHub. In the provided source code example, a directory named “Endpoints” is designated to house all endpoint-related components. However, feel free to adopt any directory structure that aligns with your project requirements. Don’t hesitate to reach out to us for the GitHub link.
Wrapping Up
In this article, we delved into the construction of a .NET 7 Web API project, emphasizing service-controller separation and the differentiation between entity models and view models. By embracing this architectural paradigm, we enhance the separation of concerns, bolster maintainability, and imbue our project with greater flexibility. The service layer serves as a repository for business logic, interfacing with the repository layer, while the controller layer manages API requests and responses, mediating data transformations between entity models and view models. Through the adoption of this architectural framework, we can develop resilient and scalable APIs tailored to our application requirements.
Following the outlined steps in this article empowers you to implement a well-structured and sustainable .NET 7 Web API project. By adhering to service-controller separation and maintaining a clear distinction between entity models and view models, you foster improved code organization, delineation of responsibilities, and scalability. This architectural approach facilitates ease of maintenance and future project expansion, ensuring the longevity and adaptability of your application.
Happy Reading!