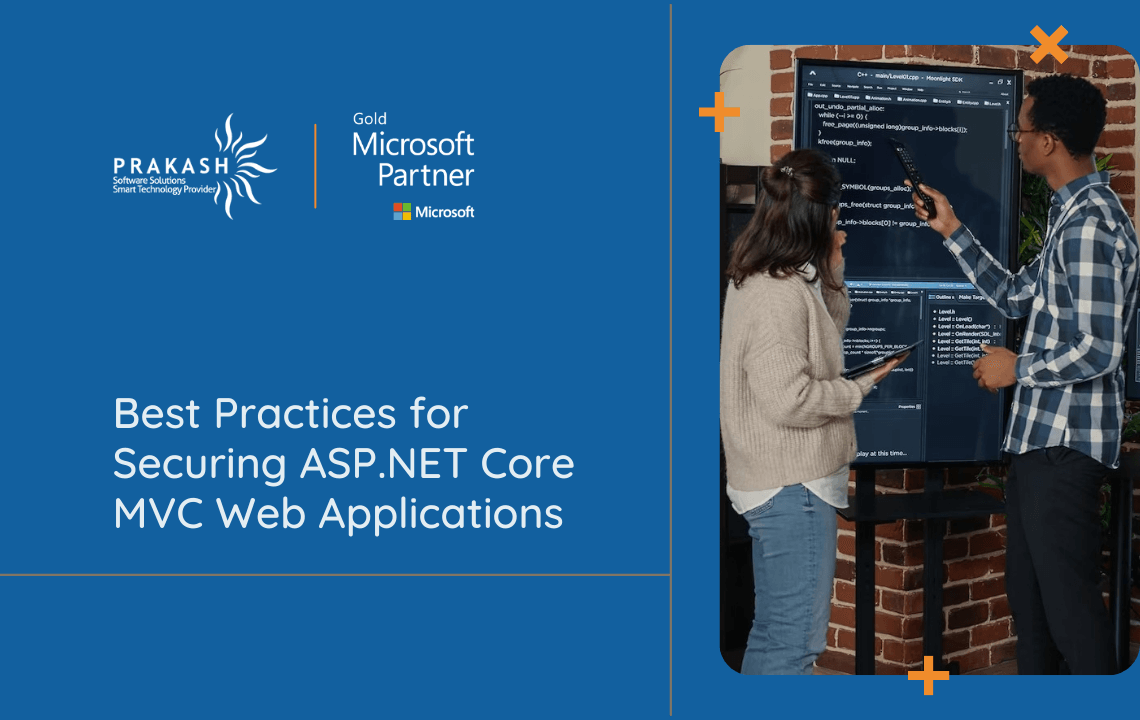
Best Practices for Securing ASP.NET Core MVC Web Applications
ASP.NET Core MVC is esteemed for web development, yet security remains vital for developers. Protecting your application against potential threats is paramount. Following best practices is crucial for improving the security of ASP.NET Core MVC applications.
This article explores various strategies and methods to bolster the security of your ASP.NET Core MVC application. Furthermore, we will discuss the significance of partnering with an ASP.NET Core development company and ASP NET MVC development company to address your application’s development requirements effectively.
Let’s begin:
Use HTTPS
Incorporating HTTPS is vital to guarantee the secure transfer of data between the client and server, minimizing the dangers linked with exposing sensitive information and thwarting potential man-in-the-middle attacks. The process of integrating HTTPS into your ASP.NET Core MVC application involves installing an SSL certificate and configuring the server accordingly. The following code snippet demonstrates how to configure HTTPS within your application:
Key points to note from the above configuration:
UseUrls: Defines the HTTPS URL for the application. Customize it to suit your requirements.
UseKestrel: Configures Kestrel, the web server utilized by ASP.NET Core, to listen on the specified port (5001 in this example) and utilize HTTPS. Ensure to update the port number as needed.
UseHttps: Specifies the path to the SSL certificate file (path/to/ssl/certificate.pfx) and its corresponding password (certificate-password). Substitute these placeholders with the actual file path and password for your SSL certificate.
By incorporating these configurations into your application, as exemplified, it will be securely served over HTTPS via the specified URL. Remember to substitute the placeholder values with the pertinent paths and passwords corresponding to your SSL certificate.
Utilize Authentication and Authorization
Authentication and authorization play pivotal roles in delineating access to sensitive data and features within your application. Hiring a good ASP.NET MVC development company can help you. Employ ASP.NET Core Identity to manage user authentication and authorization seamlessly. Additionally, employ role-based authorization to control access to distinct sections of your application.
To fulfill this endeavor, adhere to the following requirements:
Install the necessary NuGet packages:
- AspNetCore.Identity
- AspNetCore.Authentication.Cookies
- Update the ConfigureServices method:
- Amend the ConfigureServices method in the Startup.cs file to configure ASP.NET Core Identity and authentication.
Develop a Login Action
In the AccountController.cs file, establish a Login action to manage user login functionalities.
Controllers or action methods
Apply [Authorize] Attribute to Controllers or Action Methods
Mark the controllers or specific action methods necessitating authorization with the [Authorize] attribute.
In the provided code snippet, ASP.NET Core Identity is configured, and cookie-based authentication is established. The Login action within the AccountController effectively manages user login functionalities via SignInManager. Additionally, the [Authorize] attribute is employed to restrict access to the AdminController and its associated actions.
By adhering to these procedures, you can seamlessly integrate authentication and authorization into your ASP.NET Core application utilizing ASP.NET Core Identity. It’s imperative to tailor the code to meet your specific requirements and user management prerequisites. Connecting with a good ASP NET Core development company can ease the process.
Enhance Security with Strong Passwords
Encourage users to create robust passwords and enforce password policies to ensure their strength. Employ password hashing techniques to securely store passwords within your application. Furthermore, consider implementing two-factor authentication to fortify security measures.
To enact these enhancements, modify the ConfigureServices method within the Startup.cs file to configure ASP.NET Core Identity and incorporate password policies.
Revise the ConfigureServices Method
Amend the ConfigureServices method within the Startup.cs file to incorporate password hashing configurations.
Create a Custom Password Validator
Optionally, develop a custom password validator by crafting a class that inherits from IPasswordValidator<IdentityUser> and overrides the ValidateAsync method.
Integrate Two-Factor Authentication
Enhance the security protocols of your application by introducing two-factor authentication. For detailed guidance on setting up and integrating two-factor authentication, consult the official ASP.NET Core documentation provided at: ASP.NET Core 2FA Documentation.
By adhering to these recommendations, you can enforce strong password policies and ensure secure password management within your ASP.NET Core application. Moreover, you retain the flexibility to incorporate a personalized password validator and deploy two-factor authentication to reinforce the application’s security stance.
Ensure User Input Validation
Prioritize the validation of user input to thwart potential security vulnerabilities like cross-site scripting (XSS) and SQL injection attacks. Leverage ASP.NET Core’s built-in model validation or leverage third-party validation libraries for comprehensive input validation.
Begin by creating a model class that encompasses properties representing the user input fields. Subsequently, apply validation attributes to these model properties to enforce desired validation rules.
In the provided example, we introduce a UserInputModel class featuring properties denoting user input fields such as Username, Email, and Password. To enforce validation rules on these properties, we employ validation attributes such as [Required], [StringLength], and [EmailAddress].
Subsequently, within the controller, employ the ModelState.IsValid property to assess the validity of the model. If the model fails validation, respond accordingly by returning an appropriate response or displaying relevant error messages.
In the provided scenario, the UserController features a CreateUser action that accepts a UserInputModel as a parameter. We utilize ModelState.IsValid to validate the model’s integrity. If the model fails validation, a bad request response is returned, accompanied by the ModelState object containing pertinent error information. Conversely, if the model passes validation, user creation proceeds as planned.
By integrating validation attributes into your model properties and leveraging ModelState.IsValid, you uphold stringent validation standards aligned with predefined rules. This strengthening helps reduce the likelihood of common security threats like cross-site scripting (XSS) and SQL injection attacks. Tailor the validation attributes and error handling mechanisms to match your unique validation requirements and standards.
For added security measures, contemplate integrating security headers such as Content Security Policy (CSP), X-Frame-Options, and X-XSS-Protection. These headers act as robust safeguards against prevalent web application vulnerabilities like cross-site scripting and clickjacking attacks.
Make sure to include the Microsoft.AspNetCore.Mvc.RazorPages package in your project to facilitate these enhancements. If absent, install it promptly.
Navigate to the Startup.cs file and augment the ConfigureServices method with the following code snippet to enable Razor Pages and configure the desired security headers.
In the aforementioned example, we integrate middleware into the ASP.NET Core pipeline via the app. Use method. Within this middleware, we configure the following security headers:
Content-Security-Policy: Specifies permitted sources for various content types. In the example, ‘self’ is set to allow content only from the same origin. Customization of this policy is feasible to meet specific requirements.
X-Frame-Options: Ensures prevention of website embedding in iframes from different domains. By setting it to SAMEORIGIN, embedding is restricted to the same origin.
X-XSS-Protection: Activates the browser’s built-in Cross-Site Scripting (XSS) protection. The setting 1; mode=block directs the browser to block the response upon detection of an XSS attack.
Incorporating this middleware ensures inclusion of the specified security headers in the response to every request made to your ASP.NET Core application. This proactive measure serves to safeguard against common web application vulnerabilities such as cross-site scripting (XSS) and clickjacking attacks.
Introduce Rate Limiting
To mitigate risks associated with denial-of-service attacks and brute-force attacks, consider implementing rate limiting. This can be achieved through ASP.NET Core middleware or via a third-party library.
To implement rate limiting, follow these steps:
Install the AspNetCoreRateLimit NuGet package in your ASP.NET Core project.
Open the Startup.cs file and insert the following code within the ConfigureServices method to configure rate limiting.
Incorporate Rate-Limiting Configuration
Include the rate-limiting configuration within your appsettings.json file.
In the provided example, rate limiting is configured using the IpRateLimitOptions and IpRateLimitPolicies sections within the appsettings.json file. This setup permits 100 requests per minute for all endpoints. You have the flexibility to tailor the rate-limiting rules and policies to align with your specific requirements.
By integrating rate limiting, you can effectively thwart denial-of-service (DoS) attacks and brute-force attacks by restricting the number of requests permitted within a designated timeframe. The AspNetCoreRateLimit package offers a straightforward solution for implementing rate limiting within ASP.NET Core applications.
Employing a Web Application Firewall (WAF)
An ASP.NET Core development company is well aware of the pros and cons. With their skilled and robust team, it can help you. Enhance the security measures of your application by implementing a Web Application Firewall (WAF) to safeguard against threats such as SQL injection, cross-site scripting, and cross-site request forgery (CSRF). While leveraging a WAF significantly improves application security, its deployment typically involves utilizing a third-party solution or a cloud-based service.
To set up a WAF in Azure Portal, log in and create an Azure Web Application Firewall (WAF) resource. Next, configure WAF rules to align with your application’s security requirements. For detailed instructions on configuring the Azure WAF, refer to the Azure documentation.
Once the Azure WAF setup is complete, integrate it with your ASP.NET Core application by using an Azure Application Gateway. This involves creating an Application Gateway resource and associating it with the backend pool of your application.
To complete the integration, update the Startup.cs file in your ASP.NET Core application to enable HTTPS redirection and utilize the Azure Application Gateway as a reverse proxy. This systematic approach ensures robust protection against various web-based threats, thereby strengthening the security posture of your ASP.NET Core application.
In the previous example, we introduce the app.UseHttpsRedirection() middleware to seamlessly redirect HTTP requests to their secure HTTPS counterparts. Subsequently, the app.UseAzureAppGateway() middleware is employed to efficiently manage requests routed through the Azure Application Gateway.
It’s imperative to acknowledge that the specifics of implementation may vary contingent upon the chosen WAF solution or service. The provided illustration elucidates the integration of the Azure WAF with an ASP.NET Core application utilizing Azure Application Gateway as a reverse proxy.
Maintain Software Vigilance
Ensure all software and libraries utilized within your application are consistently updated with the latest security patches and updates. This proactive measure encompasses the ASP.NET Core framework, any third-party libraries, and the underlying server operating system.
Sustaining the up-to-dateness of software and libraries is paramount to bolstering the security posture of your ASP.NET Core application. Below are code examples and recommended practices to facilitate the maintenance of software relevance:
Update the ASP.NET Core Framework
Regularly updating the ASP.NET Core framework is pivotal for availing security patches, bug fixes, and new features. To effectuate an update, navigate to your project file (e.g., .csproj) and adjust the <TargetFramework> element to reflect the desired version. For instance, to transition to ASP.NET Core 5.0.
Keep NuGet Packages Current
Consistently maintain the currency of your NuGet packages, encompassing third-party libraries integrated into your application. Employ either the NuGet Package Manager within Visual Studio or the command-line interface (CLI) to execute package updates. For instance, to bring a package named “SomePackage” up to date with the latest version.
Implement Dependency Monitoring
Consider employing a dedicated tool or service for dependency monitoring, enabling you to receive notifications concerning new package versions and potential security vulnerabilities. Services like NuGet Vulnerability Advisor or third-party tools offer effective dependency monitoring and management capabilities.
Maintain Server Operating System Integrity
Ensure the ongoing upkeep of the server operating system that hosts your application by regularly applying the latest security patches. Consistently integrating updates provided by the operating system vendor is crucial to mitigate potential vulnerabilities effectively.
Automate Build and Deployment Processes
Implement an automated build and deployment pipeline, such as Azure DevOps or Jenkins, designed to automatically scrutinize and integrate updates during the build or deployment phases. This automated approach guarantees the utilization of the most recent software versions upon deploying your application.
By adhering to these methodologies and prioritizing software updates, you can substantially diminish security risks while ensuring your ASP.NET Core application benefits from the latest security patches, bug fixes, and enhancements.
Utilize Secure Third-Party Components
Exercise caution when incorporating third-party components and libraries into your application. Prioritize the use of secure, up-to-date components sourced from trusted repositories and vendors with established credibility.
Rely on Trusted Sources
When procuring third-party components and libraries, exclusively rely on reputable sources such as official package repositories like NuGet, well-established open-source communities, or reputable vendors known for their commitment to security and reliability.
Authenticate Component Integrity
Before integrating a third-party component, diligently verify its authenticity. Assess the author’s reputation, community feedback, and maintenance history to ensure active support and regular updates addressing security vulnerabilities.
Update Third-Party Components Regularly
Consistently update your third-party components to the latest versions to avail yourself of essential security patches and bug fixes. Utilize the NuGet Package Manager or CLI to execute updates. For instance, to update a package named “SomePackage” to its latest version.
Ensure Vigilant Vulnerability Monitoring
Safeguard against potential security risks posed by third-party components by instituting a robust monitoring system. Stay abreast of new package versions and security vulnerabilities by subscribing to security alerts or employing vulnerability monitoring tools. Consider leveraging services such as OWASP Dependency-Check or commercial solutions to diligently scan and manage dependencies for known vulnerabilities.
Uphold Stringent Code Review and Security Testing
Foster a culture of security consciousness by conducting thorough code reviews and security testing on integrated third-party components. Scrutinize these components for any discernible security vulnerabilities, misconfigurations, or inherent risks. Remain vigilant in identifying and addressing any potential security loopholes or shortcomings specific to these components.
Enforce Trust Boundaries and Rigorous Input Validation
Exercise caution when leveraging third-party components by treating them as untrusted inputs. Implement meticulous input validation and sanitization procedures to fortify your application against security vulnerabilities such as cross-site scripting (XSS) and SQL injection. Vet and sanitize data originating from these components meticulously to fortify your application’s defenses against malicious exploits.
By adhering to these methodologies, you can effectively mitigate security risks associated with third-party components and libraries integrated into your ASP.NET Core application. By maintaining a stringent update regimen, verifying component authenticity, and instituting a secure development and integration framework, you can bolster the security and reliability of your application.
Employ Security Testing Protocols
Integrate comprehensive security testing protocols into your development workflow to identify and rectify vulnerabilities and weaknesses. Utilize specialized tools such as penetration testing and vulnerability scanning to identify potential security lapses and vulnerabilities within your ASP.NET Core application.
Implementing robust security testing measures within your ASP.NET Core application is critical for identifying and addressing potential vulnerabilities and weaknesses. While providing code examples for penetration testing and vulnerability scanning is beyond the scope of this context, I can offer general guidance on how to approach security testing within your application.
Penetration Testing: Engage security professionals or ethical hackers to conduct simulated attacks against your application, aiming to uncover vulnerabilities. Techniques may include manual testing of user input fields for common vulnerabilities like SQL injection and cross-site scripting (XSS), assessment of authentication mechanisms, and evaluation of API security.
Vulnerability Scanning: Utilize automated tools to scan your application’s codebase, configuration, and dependencies for known security vulnerabilities. Popular vulnerability scanning tools include OWASP ZAP (Zed Attack Proxy), Nessus, OpenVAS, and Burp Suite. These tools offer features such as scanning for injection attacks, XSS, or insecure configurations, along with detailed reports highlighting identified vulnerabilities.
Promote Secure Coding Practices
Embrace secure coding practices from inception to minimize vulnerabilities. This encompasses thorough validation and sanitization of user input to thwart common threats like SQL injection and XSS, robust authentication and authorization mechanisms, deployment of parameterized queries or ORMs to counter SQL injection, adoption of secure session management practices, and meticulous maintenance of software updates for your application and its dependencies to address security vulnerabilities effectively.
Remember, security testing is an ongoing endeavor and should be performed regularly throughout the development lifecycle and after significant modifications to your application. While I cannot provide specific code examples for penetration testing or vulnerability scanning, embedding these practices within your development process will aid in identifying and rectifying security issues within your ASP.NET Core application.
In summary, by adhering to the aforementioned best practices, you can significantly bolster the security and integrity of your ASP.NET Core MVC application. It’s imperative to acknowledge that security is an ongoing endeavor requiring continuous vigilance and adaptation to evolving threats. Thus, staying current with security threats and updates is paramount for safeguarding your application effectively. Connect with a good ASP.NET MVC development company and reap good ROI.
Happy Reading!