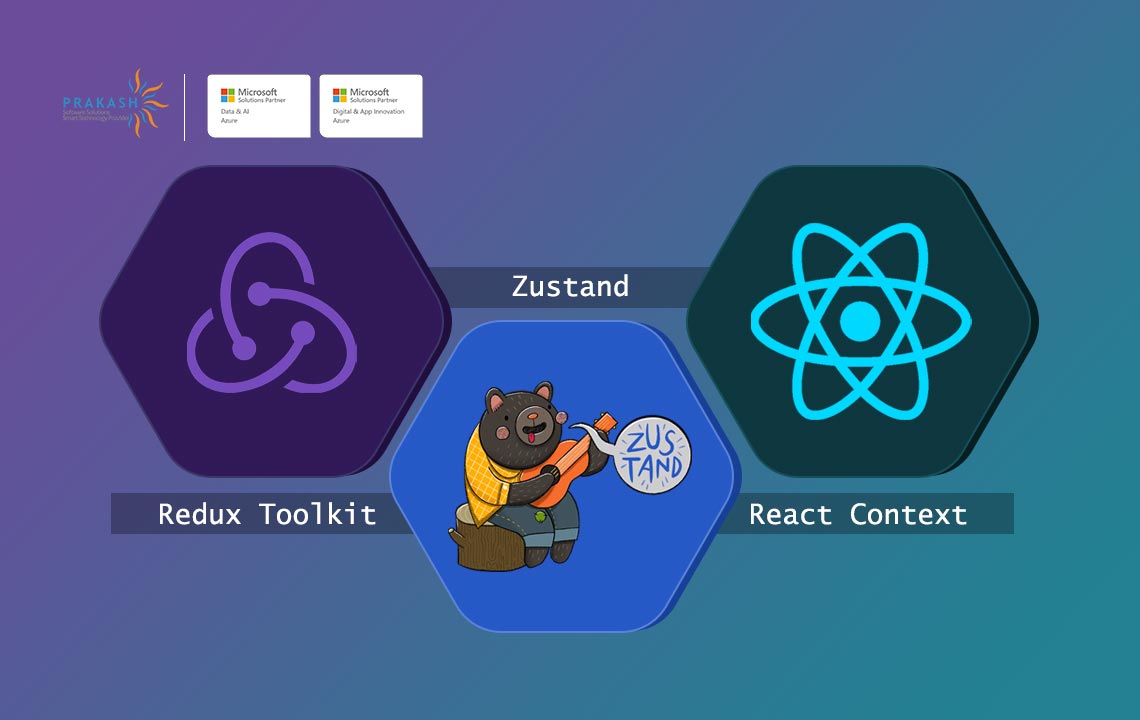
State Management: Comparing Redux Toolkit, Zustand, and React Context
State management is an essential feature of modern web development, especially in complex systems. Three popular state management choices for React projects are Redux, Zustand, and Context API; each has pros, cons, and best practices. This blog post will compare these tools, focusing on their advantages, drawbacks, and practical applications.
State Management in React
React employs a unidirectional data flow, in which props are used to transfer state from parent to child components. However, using props to manage state gets difficult when an application grows in size. Typical difficulties include the following:
Prop drilling (moving through several component levels)
The challenge of upholding a global state
Performance problems brought on by pointless re-renders
The difficulty of controlling adverse effects
By providing centralized or lightweight options to effectively handle shared state, state management libraries offer systematic answers to these problems.
Redux Toolkit
For state management in React, Redux has long been a favorite. Redux was made simpler with the release of Redux Toolkit (RTK), which included user-friendly APIs and reduced boilerplate code.
Advantages
- Centralized state management: Redux makes it simple to maintain and retrieve data by storing the application state in a single store.
- Predictable state changes: Redux makes testing and debugging easier by adhering to a rigid unidirectional data flow.
- Strong community support: Redux is surrounded by a sizable community as well as a multitude of libraries and tools.
Drawbacks
Boilerplate code: Writing a lot of boilerplate code is a common part of Redux, which could lengthen development time.
Steeper learning curve: Redux’s principles, including reducers and actions, may be difficult for beginners to understand at first.
Example: Counter Application with Redux Toolkit
Zustand
Zustand is a performance and simplicity-focused minimalist state management library. Zustand is simpler and lighter than Redux because it doesn’t require actions, reducers, or middleware.
Advantages
- Minimal setup: Zustand is quicker and simpler to set up than Redux since it uses less boilerplate code.
- Lightweight: Zustand is a performance-focused tiny library that is appropriate for smaller projects.
- Simple integration: Zustand works well with other state management programs, such as MobX or Redux.
Drawbacks
- Restricted ecosystem: Zustand has fewer third-party tools and libraries since its ecosystem is smaller than Redux’s.
- Not appropriate for complicated applications: Zustand’s simplicity can make it less appropriate for intricate and sizable applications.
Example: Counter Application with Zustand
React Context
A feature of React that allows data sharing between components without prop digging is React Context. It works best for localized or small-scale state administration.
Advantages
- Built-in React feature: React includes the Context API, thus no further libraries or dependencies are required.
- Simplicity: Without requiring complicated configurations, the Context API offers a simple method of sharing state between components.
- Simple to use for small applications: Context API can serve as an adequate state management solution for small-scale applications.
Drawbacks
- Performance issues: In larger applications, the context API may result in needless re-renders, which could affect performance.
- Global state management: Tracking state changes may become difficult if you use the Context API for global state management.
Example: Counter Application with React Context
Tool | Best For | Examples |
---|---|---|
Redux Toolkit | Large-scale applications with complex state and asynchronous requirements. | E-commerce sites, enterprise dashboards. |
Zustand | Small to medium-sized projects where simplicity and performance are priorities. | Personal projects, lightweight apps. |
React Context | Localized state or small applications with minimal state management needs. | Theme togglers, language selectors. |
By 2025, developers will have access to a wide range of state management solutions designed to meet various requirements. Redux Toolkit shines for its ecosystem and scalability, Zustand is a lightweight yet efficient alternative, and React Context is best suited for small-scale projects.
The choice is determined by your project’s size, complexity, and performance requirements. See which of these tools best suits your development process by giving them a try!