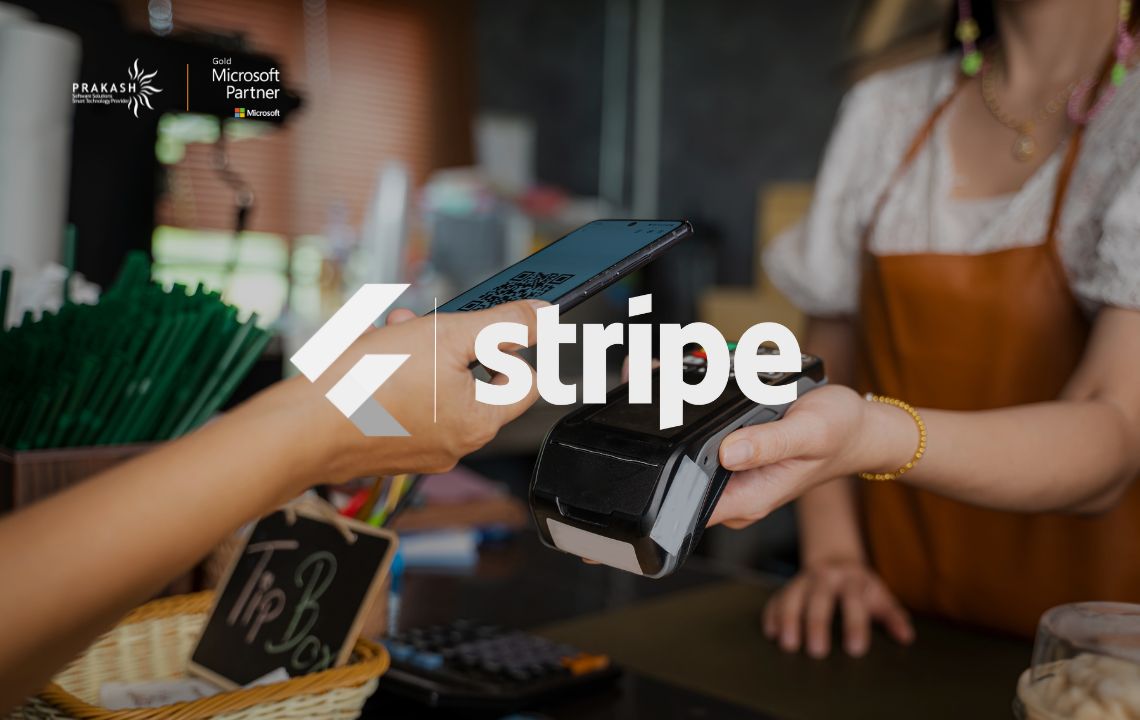
Flutter – Integrate Stripe Payment Gateway
In the world of app development, smooth transactions depend on the integration of a reliable and effective payment gateway.
Stripe integration with Flutter is an excellent choice for companies looking for Flutter development services in the USA.
With support for more than 135 countries and numerous currencies, Stripe is a flexible platform that makes online transactions easier across a range of applications and websites.
International firms frequently use it because of its extensive reporting, user-friendly design, and security.
Now let’s begin with
Step-by-Step Implementation
Step 1: Create a stripe account and get publishable and secret key from it
To get started, we would need to generate an API key from Stripe. To do this, you would need to create a Stripe account. After this, login to your dashboard, activate Test mode for the purpose of integration and testing and go to Developers > API Keys to reveal your API keys (Publishable and Secret Keys).
Step 2: Add the flutter_stripe and http package.
Add the following package to your pubspec.yaml file.
flutter_stripe: ^10.1.1 dio: ^5.4.3+1
NOTE: The version may change depending on your usage. We have preferred this package because it is developed by stripe itself and it is well maintained project with error free code.
Step 3: Set the publishable key in void main function.
Map<String, dynamic>? paymentIntent;
void main () {
//Initialize Flutter Binding
WidgetsFlutterBinding.ensureInitialized();
//Assign publishable key to flutter_stripe
Stripe.publishableKey = '<your_publishable_key>';
runApp(const MyApp());
}
This is our Make Payment function:
Future<void> makePayment() async {
try {
//STEP 1: Create Payment Intent
paymentIntent = await createPaymentIntent('100', 'USD');
//STEP 2: Initialize Payment Sheet
await Stripe.instance
.initPaymentSheet(
paymentSheetParameters: SetupPaymentSheetParameters(paymentIntentClientSecret: paymentIntent!['client_secret'], style: ThemeMode.light, merchantDisplayName: 'Ikay'))
.then((value) {});
//STEP 3: Display Payment sheet
displayPaymentSheet();
} catch (err) {
throw Exception(err);
}
}
Step 4: Add 1 button from where a bottom sheet for payment will open.
We will add 1 elevated button in screen with On Tap Function which will open the payment page and we can handle the different case like payment is successful or cancelled. Let’s learn step by step process to open the payment sheet and handle different scenarios.
- Payment intent data via http request:
We Start by creating payment intent by defining a createPaymentIntent function that takes the amount we’re paying and the currency.
createPaymentIntent(String amount, String currency) async {
Dio dio = Dio();
Response response = await dio.post(
'https://api.stripe.com/v1/payment_intents',
options: Options( headers: {
'Authorization': 'Bearer
sk_test_51PU1tb08kFrd3w5s8LLZs9DJGIx7800CYYvMkSCOFwbfmAolplKg2UP
whXI0IIiqJfVAJewCnrKqBvckcfe4ck4x00g9Z6XdYX',
'Content-Type': 'application/x-www-form-urlencoded'
}, ), data: {
'amount': amount,
'currency': currency,
},
);
if (response.statusCode == 200) {Map<String, dynamic> output = {'client_secret': response.data['client_secret'],
};
return output;
} else {
print('Error charging user: ${response.data.toString()}'); }
}
2. Display paymentsheet The final step is to display the modal sheet.
displayPaymentSheet() async {
try {
await Stripe.instance.presentPaymentSheet().then((value) {
showDialog(context: context,
builder: (_) => const AlertDialog(
content: Column(
mainAxisSize: MainAxisSize.min,
children: [
Icon(
Icons.check_circle,color: Colors.green,
size: 100.0,
),
SizedBox(height: 10.0),
Text("Payment Successful!"),
],
),
));
paymentIntent = null;
}).onError((error, stackTrace) { throw Exception(error);
});
} on StripeException catch (e) {print('Error is:---> $e');
const AlertDialog(content:Column(
mainAxisSize: MainAxisSize.min,
children: [
Row(
children: [
Icon(
Icons.cancel,color: Colors.red,
),
Text("Payment Failed"),
],
),
],
),
);
} catch (e) {print('$e');
}
}
Step 5: When the payment is successful, a confirmation message will be displayed. If the payment fails, an error message will appear.
Businesses may incorporate the Stripe payment gateway into their Flutter applications to offer a secure and seamless transaction experience by utilizing Flutter development services in the USA.
Whether you are a startup or an existing business, the functionality and user experience of your app will be improved by integrating Stripe with Flutter, which guarantees quick and easy money processing.